전역적인 예외 처리를 위해 기존 프로젝트에 Controller Advice를 도입하였다.
기존의 Controller 코드
@GetMapping("/list")
public ResponseEntity<List<UserCardDTO>> list(@AuthenticationPrincipal MemberDetailsImpl memberDetails) { // 사용자카드 리스트 가져오기
try{
return new ResponseEntity<>(userCardService.getUserCardByUserId(memberDetails.getMember().getId()), HttpStatus.OK);
} catch (RuntimeException e){
return new ResponseEntity<>(HttpStatus.INTERNAL_SERVER_ERROR);
}
}
기존에는 위와 같이 컨트롤러 기능 마다 try catch문으로 예외 처리를 진행하였다.
service에 인위적으로 예외를 발생시켜 기존 코드의 에러를 출력해보자
에러 발생 시 다음과 같은 결과를 얻게 된다.
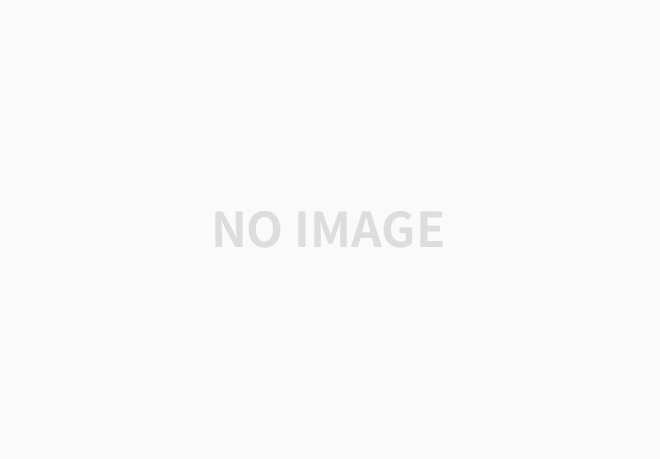
다음으로는 RestControllerAdvice가 적용하고 컨트롤러를 수정한다.
ErrorResponse
// 에러 응답 클래스
import java.util.List;
import lombok.Builder;
import lombok.Getter;
@Getter
@Builder
public class ErrorResponse {
private String code;
private String message;
private int status;
private List<String> errors;
}
GlobalExceptionHandler
// ExceptionHandler 클래스
@RestControllerAdvice
@Log4j2
public class GlobalExceptionHandler {
// 명시된 예외 이외의 모든 예외
@ExceptionHandler(Exception.class)
public ResponseEntity<ErrorResponse> handleException(Exception e) {
log.error(e);
return createErrorResponse(HttpStatus.INTERNAL_SERVER_ERROR, "SERVER_ERROR", "Internal server error occurred");
}
// 400 잘못된 요청 데이터
@ExceptionHandler(HttpMessageNotWritableException.class)
public ResponseEntity<ErrorResponse> handleHttpMessageNotWritableException(HttpMessageNotWritableException e) {
log.error(e);
return createErrorResponse(HttpStatus.BAD_REQUEST, "INVALID_REQUEST_DATA", "잘못된 요청 데이터입니다");
}
private ResponseEntity<ErrorResponse> createErrorResponse(HttpStatus status, String code, String message) {
ErrorResponse response = ErrorResponse.builder()
.code(code)
.message(message)
.status(status.value())
.build();
return new ResponseEntity<>(response, status);
}
}
Controller
@GetMapping("/list")
public ResponseEntity<List<UserCardDTO>> list(@AuthenticationPrincipal MemberDetailsImpl memberDetails) { // 사용자카드 리스트 가져오기
return new ResponseEntity<>(userCardService.getUserCardByUserId(memberDetails.getMember().getId()), HttpStatus.OK);
}
예외 발생 결과
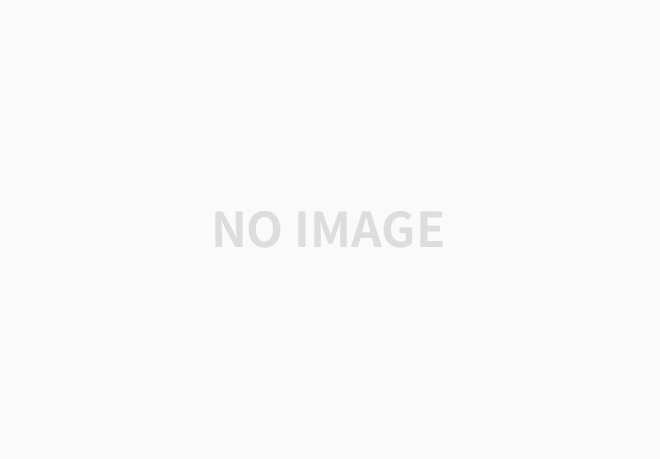
1. 코드 중복 감소
2. 비즈니스 로직과 예외 처리의 관심사 분리
3. 일관된 예외 처리
'Spring' 카테고리의 다른 글
의존관계 자동 주입 (1) | 2024.12.25 |
---|---|
Spring Security - 인증 및 권한 부여 (0) | 2024.12.16 |